Back to Portfolio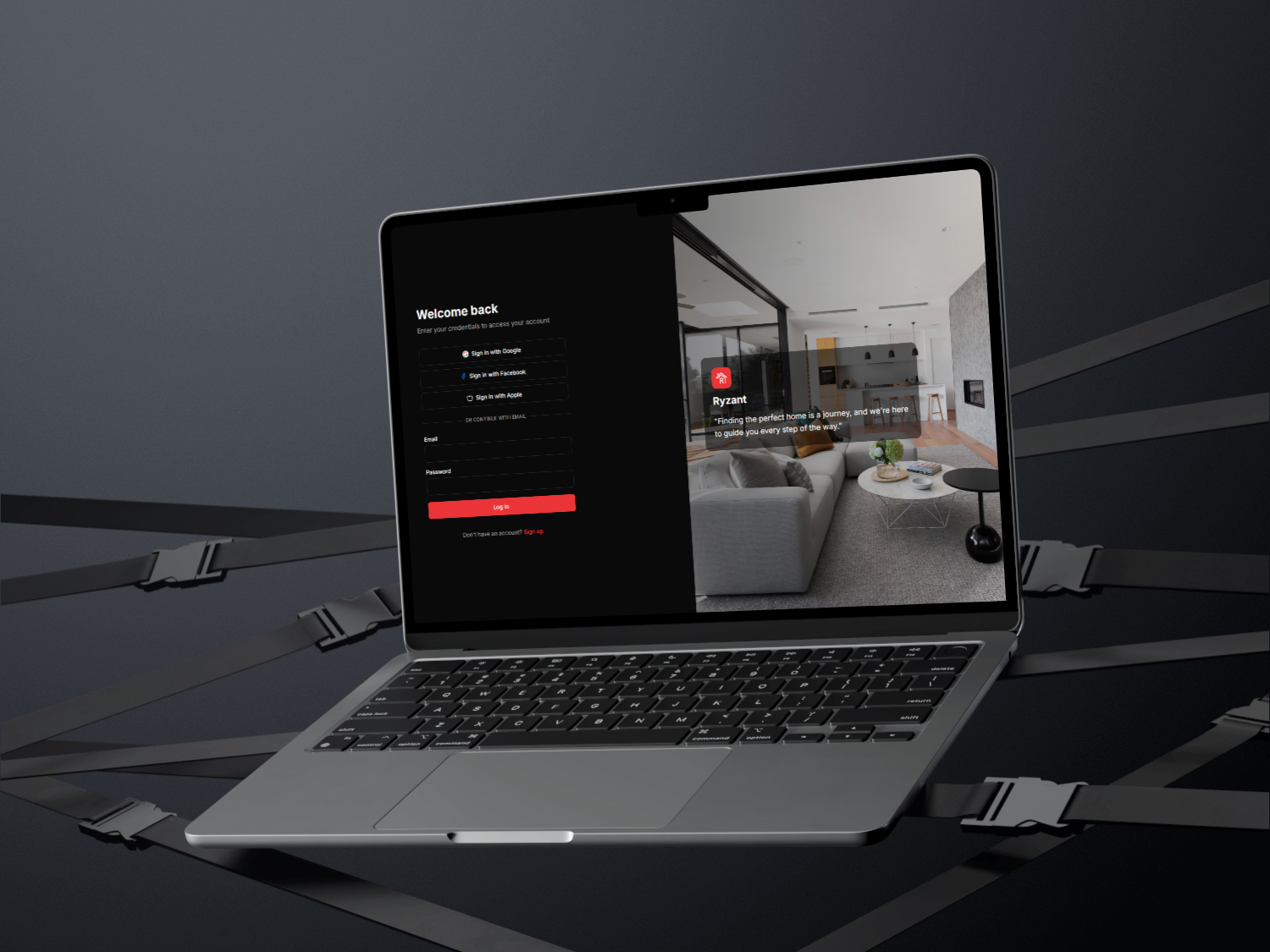
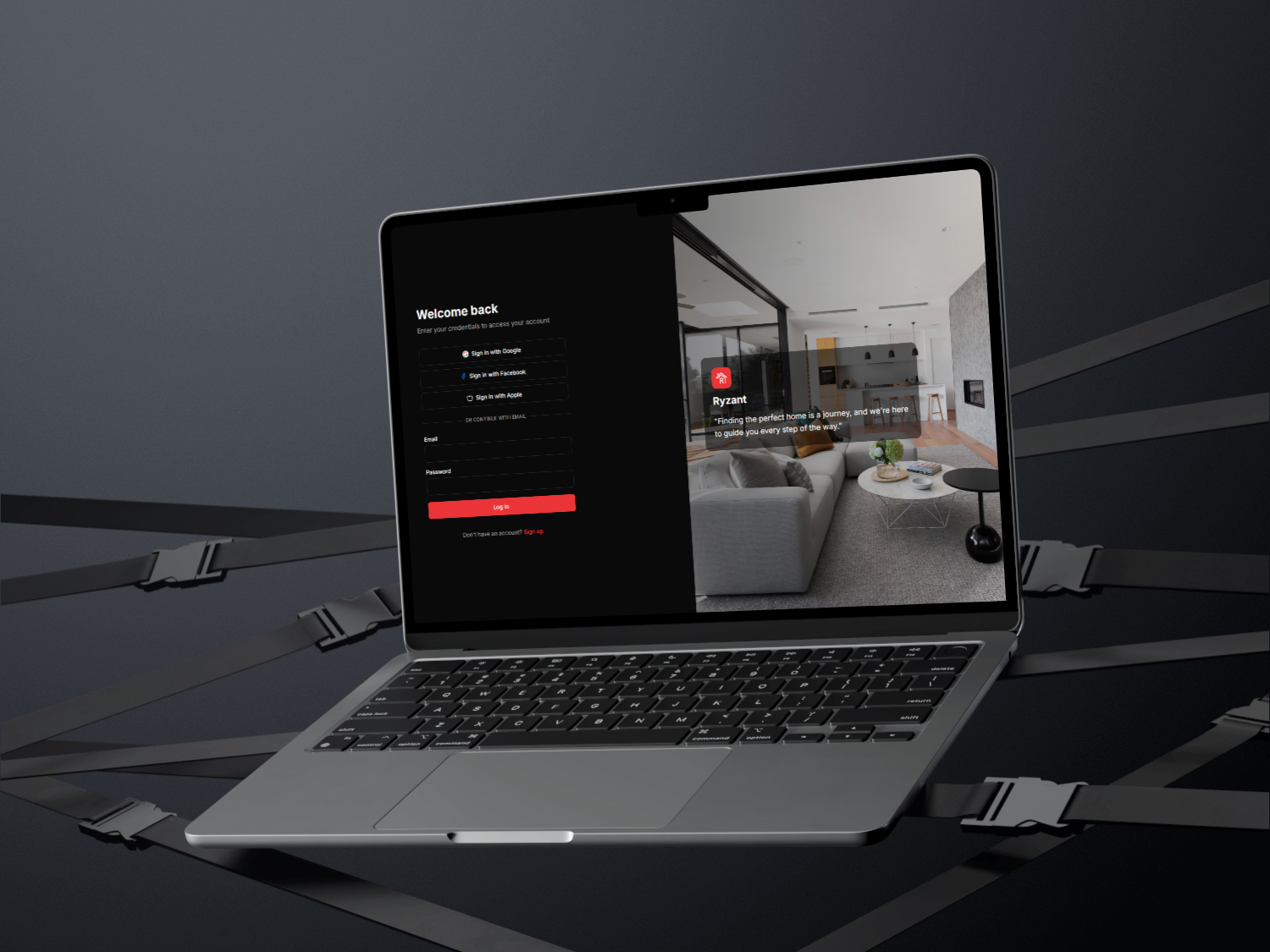
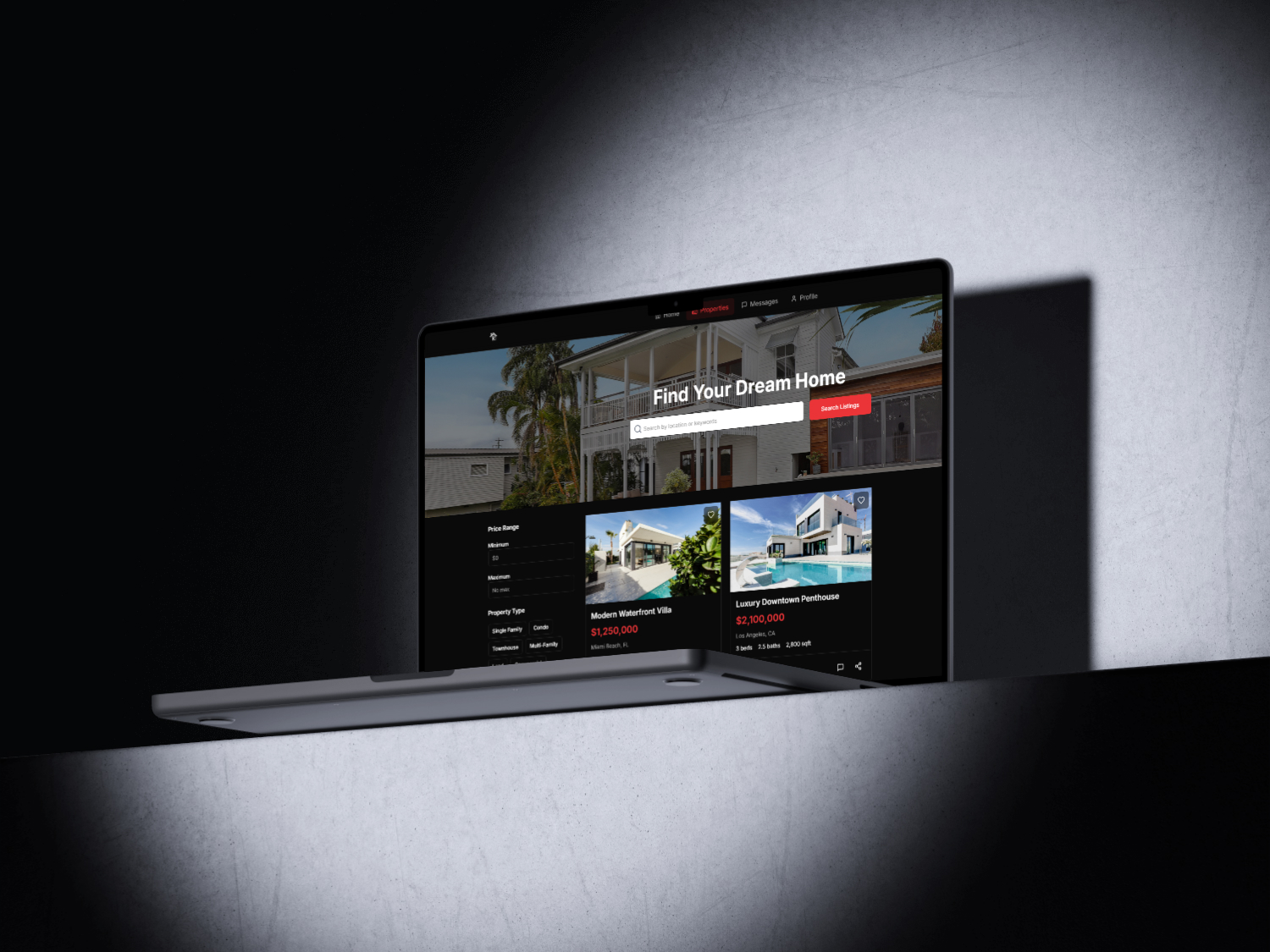
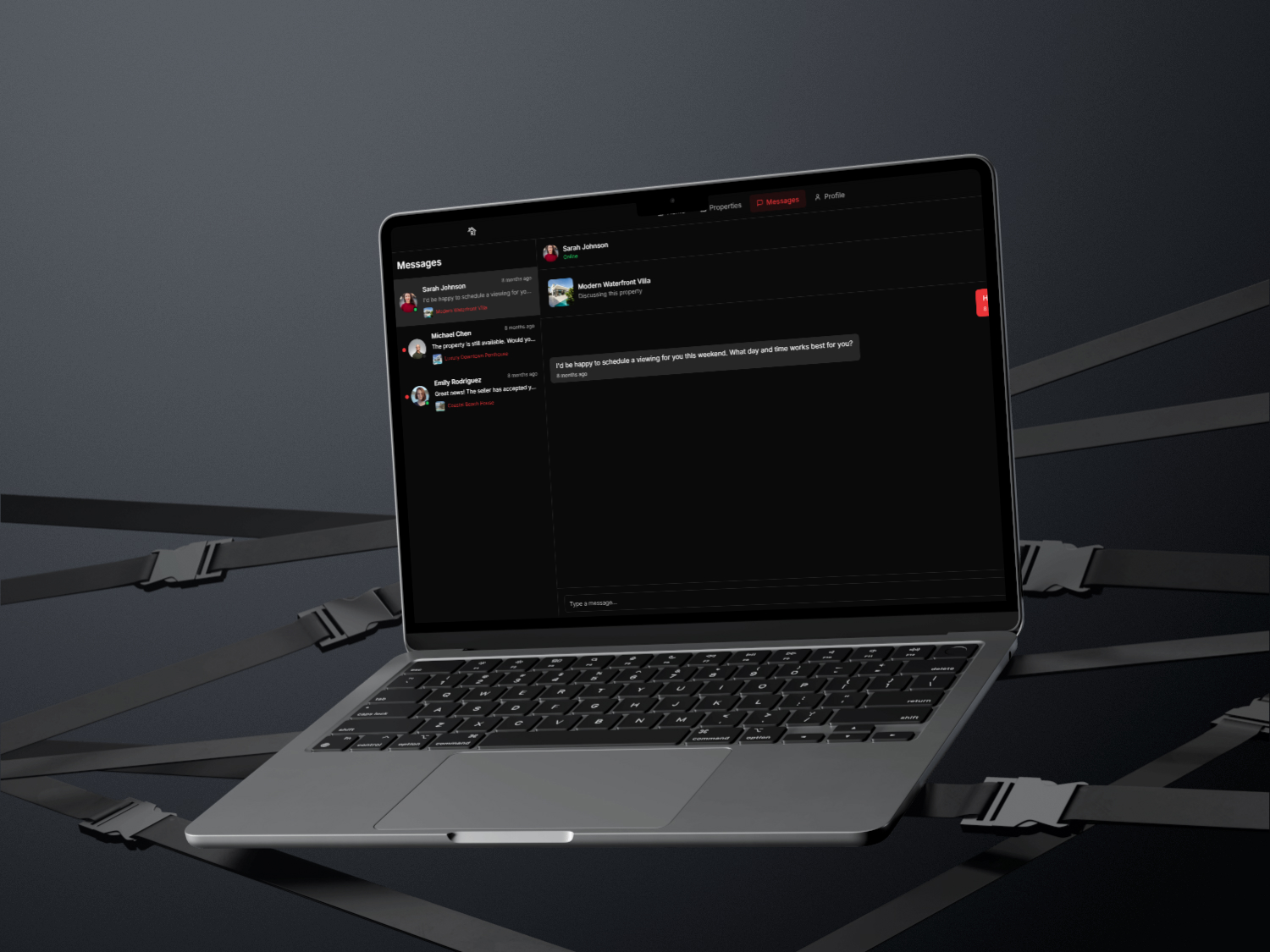
Ryzant - Modern Real Estate Platform
2024-11-27
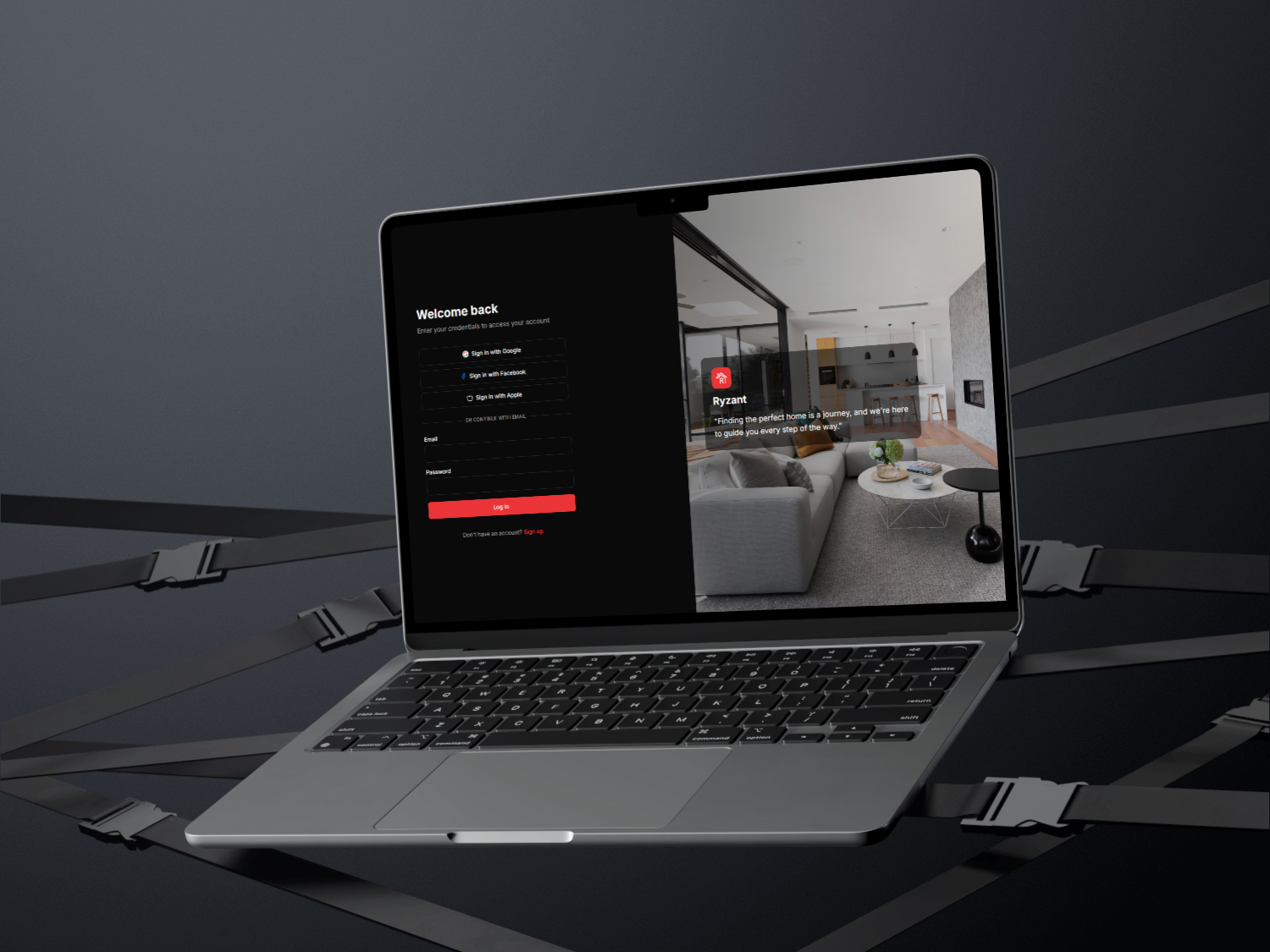
Ryzant - Modern Real Estate Platform
A sophisticated real estate platform that combines the visual appeal of Instagram with the functionality of Zillow, built specifically for realtors to showcase their property listings and connect with potential buyers.
Key Features
For Realtors
- Professional Profiles: Customizable portfolio pages with property listings
- Listing Management: Easy-to-use interface for creating and managing property listings
- Analytics Dashboard: Track engagement and interest in properties
- Messaging System: Built-in communication with potential buyers
- Tour Scheduling: Integrated calendar for property viewings
For Users
- Property Discovery: Instagram-style feed of property listings
- Advanced Search: Filter properties by location, price, features
- Saved Properties: Bookmark favorite listings
- Direct Messaging: Easy communication with realtors
- Tour Booking: Schedule property viewings in-app
Technical Implementation
The platform is built with modern web technologies and best practices:
// Example of the property listing component
interface PropertyListing {
id: string;
title: string;
price: number;
location: {
address: string;
city: string;
state: string;
zip: string;
coordinates: {
lat: number;
lng: number;
};
};
features: {
bedrooms: number;
bathrooms: number;
squareFeet: number;
yearBuilt: number;
};
images: string[];
realtor: {
id: string;
name: string;
avatar: string;
agency: string;
};
}
const PropertyCard: React.FC<{ listing: PropertyListing }> = ({ listing }) => {
const [isLiked, setIsLiked] = useState(false);
const [isSaved, setIsSaved] = useState(false);
const handleLike = async () => {
try {
await likeProperty(listing.id);
setIsLiked(true);
} catch (error) {
console.error('Error liking property:', error);
}
};
return (
<div className="property-card">
<ImageCarousel images={listing.images} />
<div className="property-details">
<h3>{listing.title}</h3>
<p className="price">${listing.price.toLocaleString()}</p>
<div className="features">
<span>{listing.features.bedrooms} beds</span>
<span>{listing.features.bathrooms} baths</span>
<span>{listing.features.squareFeet} sq ft</span>
</div>
<div className="actions">
<button onClick={handleLike}>
{isLiked ? 'Liked' : 'Like'}
</button>
<button onClick={() => setIsSaved(!isSaved)}>
{isSaved ? 'Saved' : 'Save'}
</button>
</div>
</div>
</div>
);
};
Design System
Color Palette
- Primary: #E5383B (Customizable per brand)
- Secondary: Generated complementary colors
- Neutral: Grayscale palette for UI elements
- Accent: Highlight colors for CTAs and important elements
Typography
- Headers: Space Grotesk
- Body: Inter
- Monospace: JetBrains Mono (for code elements)
Components
- Modular card system for property listings
- Reusable form components
- Interactive image carousels
- Message threads and chat bubbles
- Custom buttons and input fields
Accessibility Features
- WCAG 2.1 AA compliant
- Screen reader optimized
- Keyboard navigation support
- High contrast mode
- Scalable typography
- Alt text for all images
- ARIA labels and roles
Mobile Optimization
The platform is built with a mobile-first approach:
- Responsive layouts
- Touch-friendly interfaces
- Optimized image loading
- Native-like animations
- Offline capabilities
- PWA support
Development Process
-
Research & Planning
- Market analysis
- User interviews
- Feature prioritization
- Technical architecture design
-
Design Phase
- Wireframing
- UI/UX design
- Component library creation
- Design system documentation
-
Development
- Frontend implementation
- API integration
- Testing and optimization
- Performance tuning
-
Deployment
- CI/CD setup
- Monitoring implementation
- Analytics integration
- Documentation
Future Enhancements
- Virtual tour integration
- AI-powered property recommendations
- Advanced analytics dashboard
- Market trend analysis
- Document signing integration
- Multi-language support
Performance Metrics
- Lighthouse Score: 95+
- First Contentful Paint: < 1.5s
- Time to Interactive: < 3.5s
- Core Web Vitals compliance
- SEO optimization
Security Features
- JWT authentication
- Role-based access control
- Data encryption
- GDPR compliance
- Regular security audits
- Protected API endpoints
Project Gallery
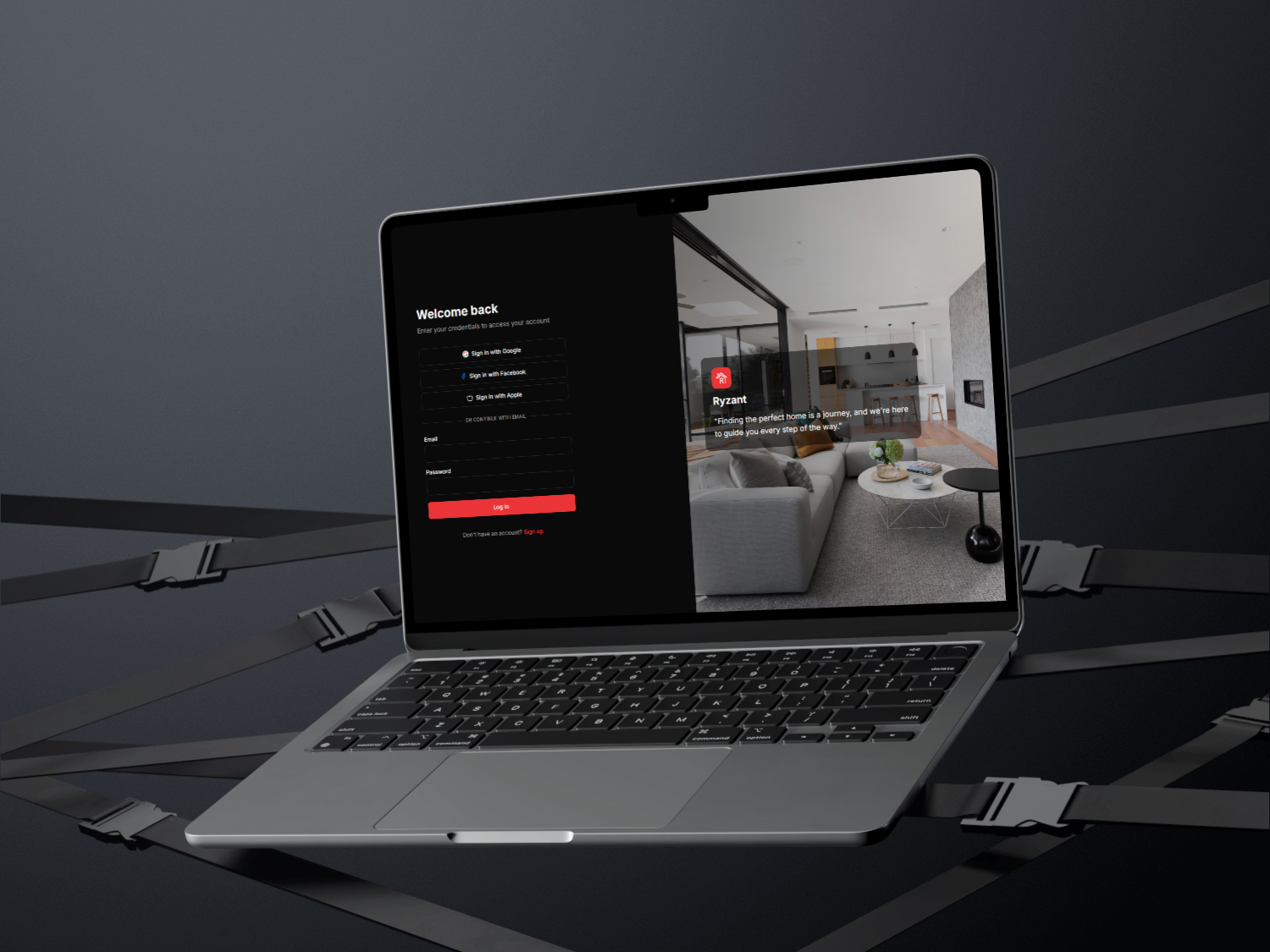
Ryzant Login
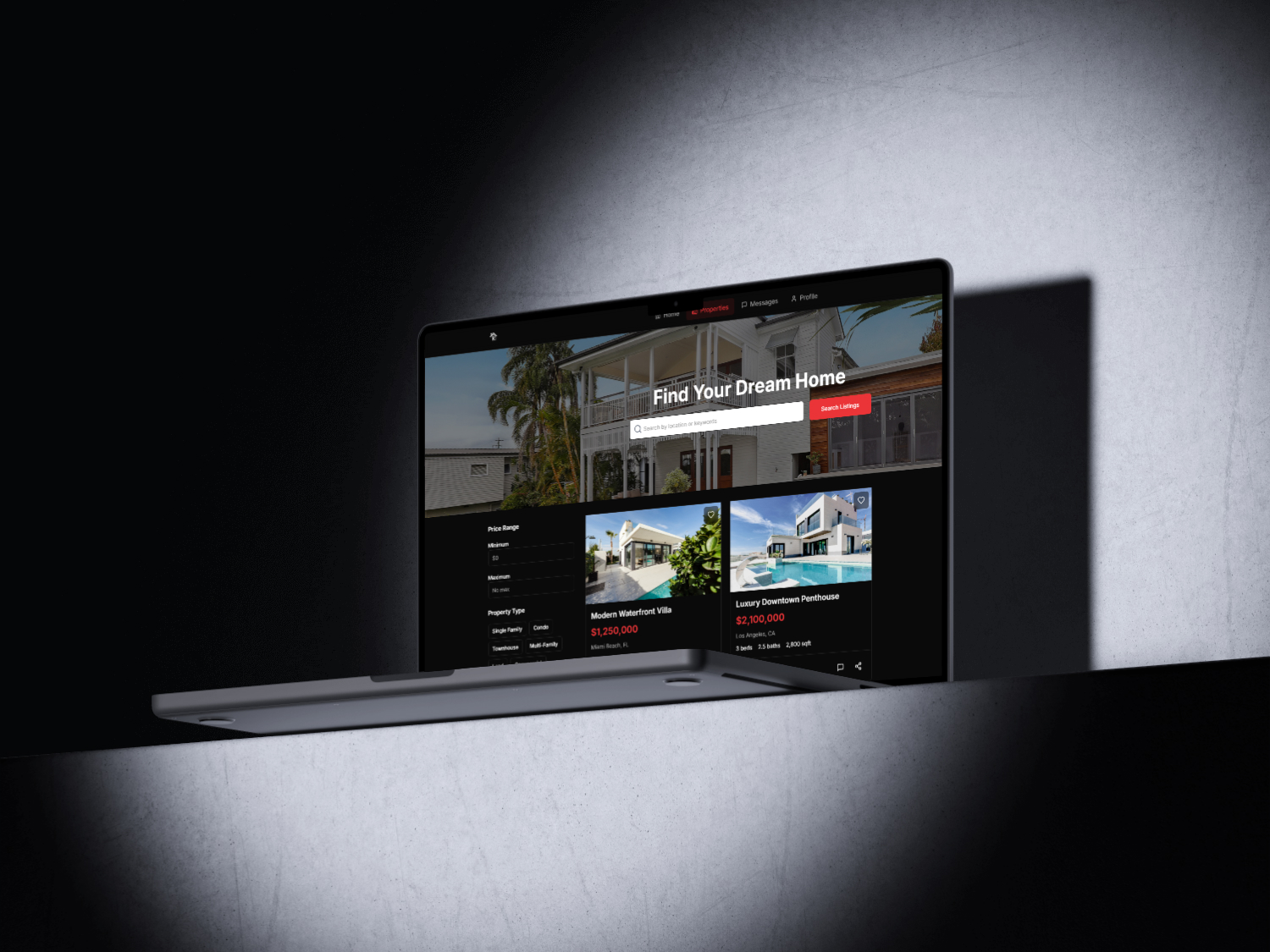
Ryzant Explore Properties
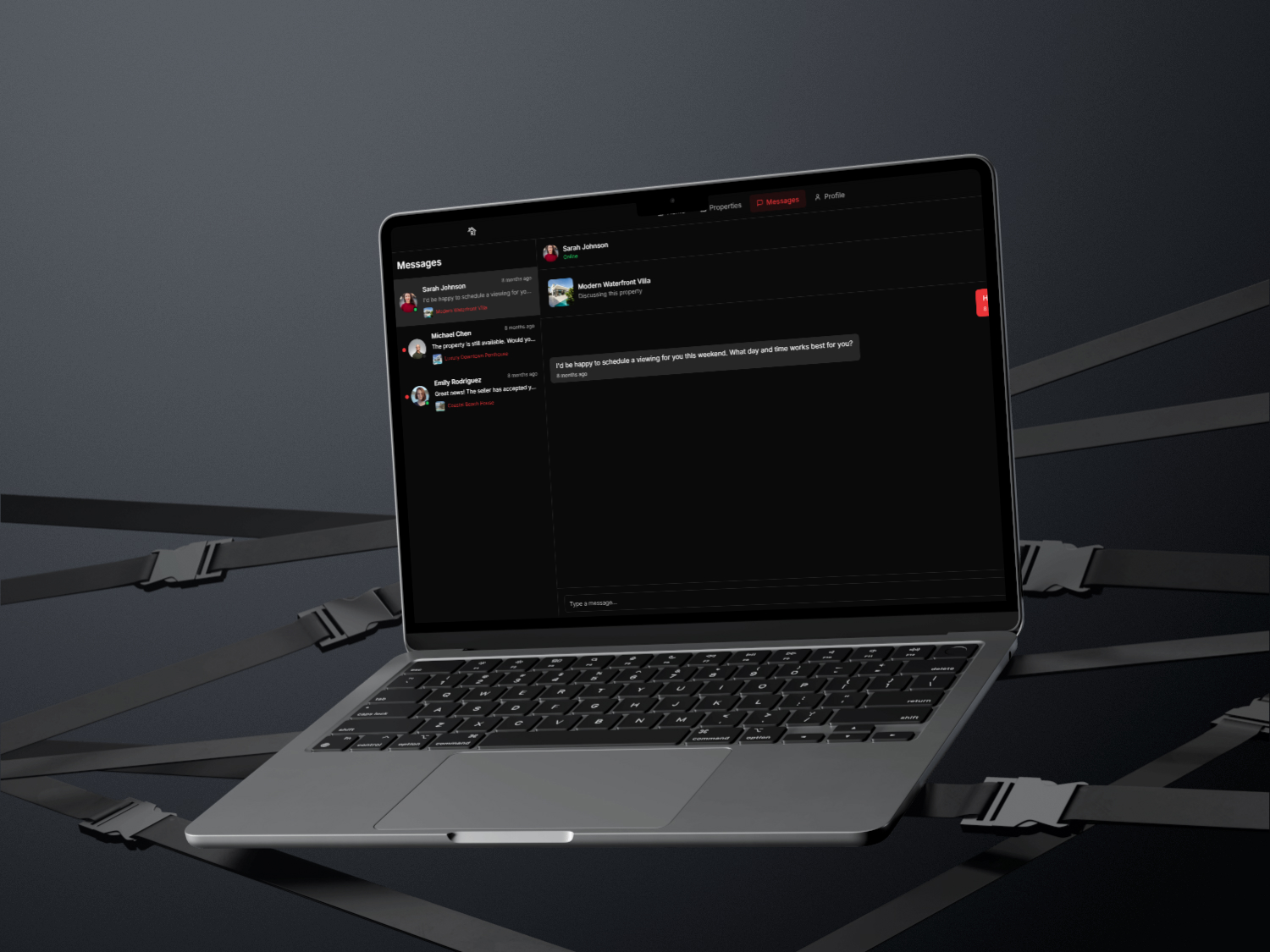
Ryzant Messaging