Back to Portfolio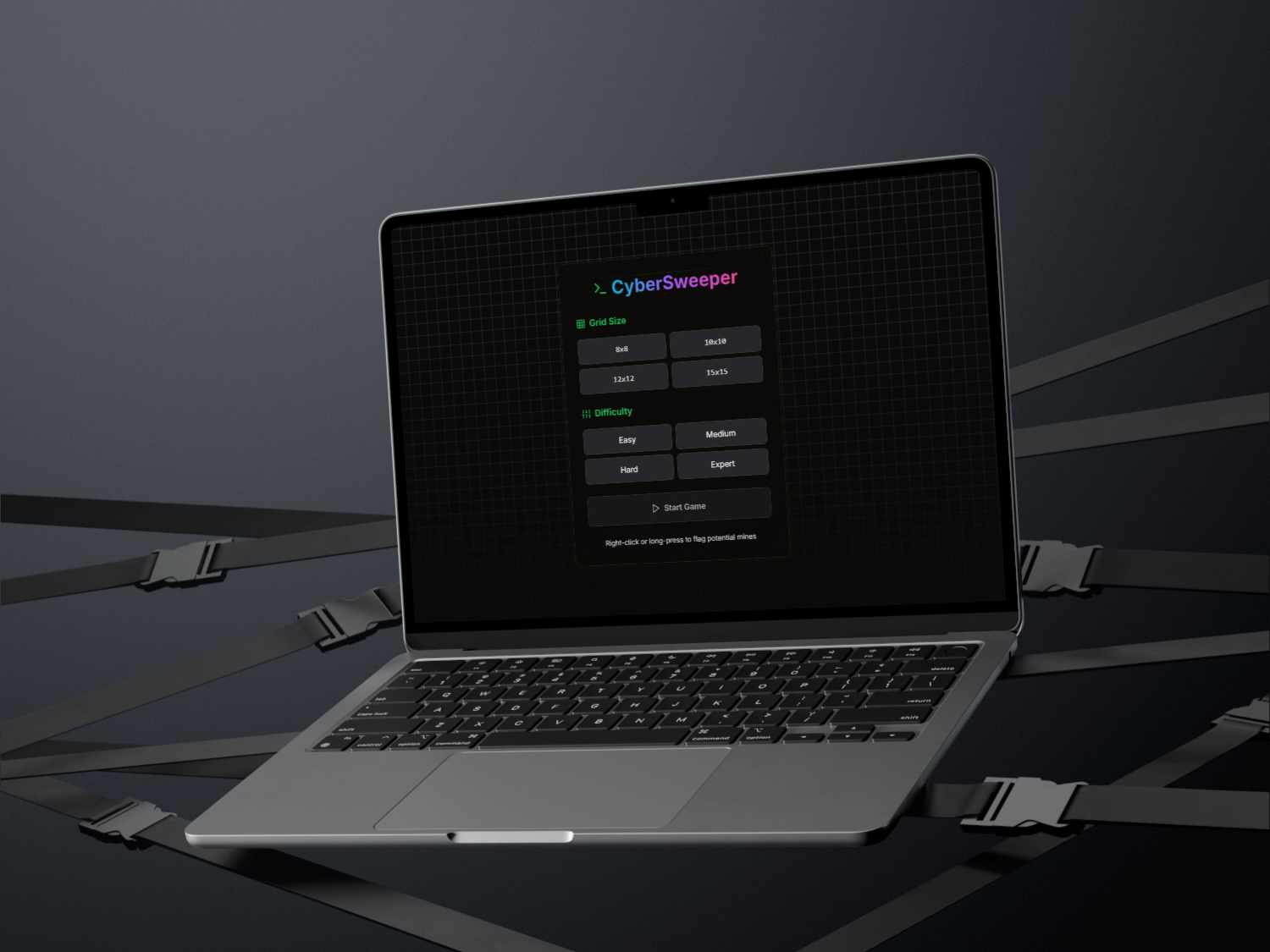
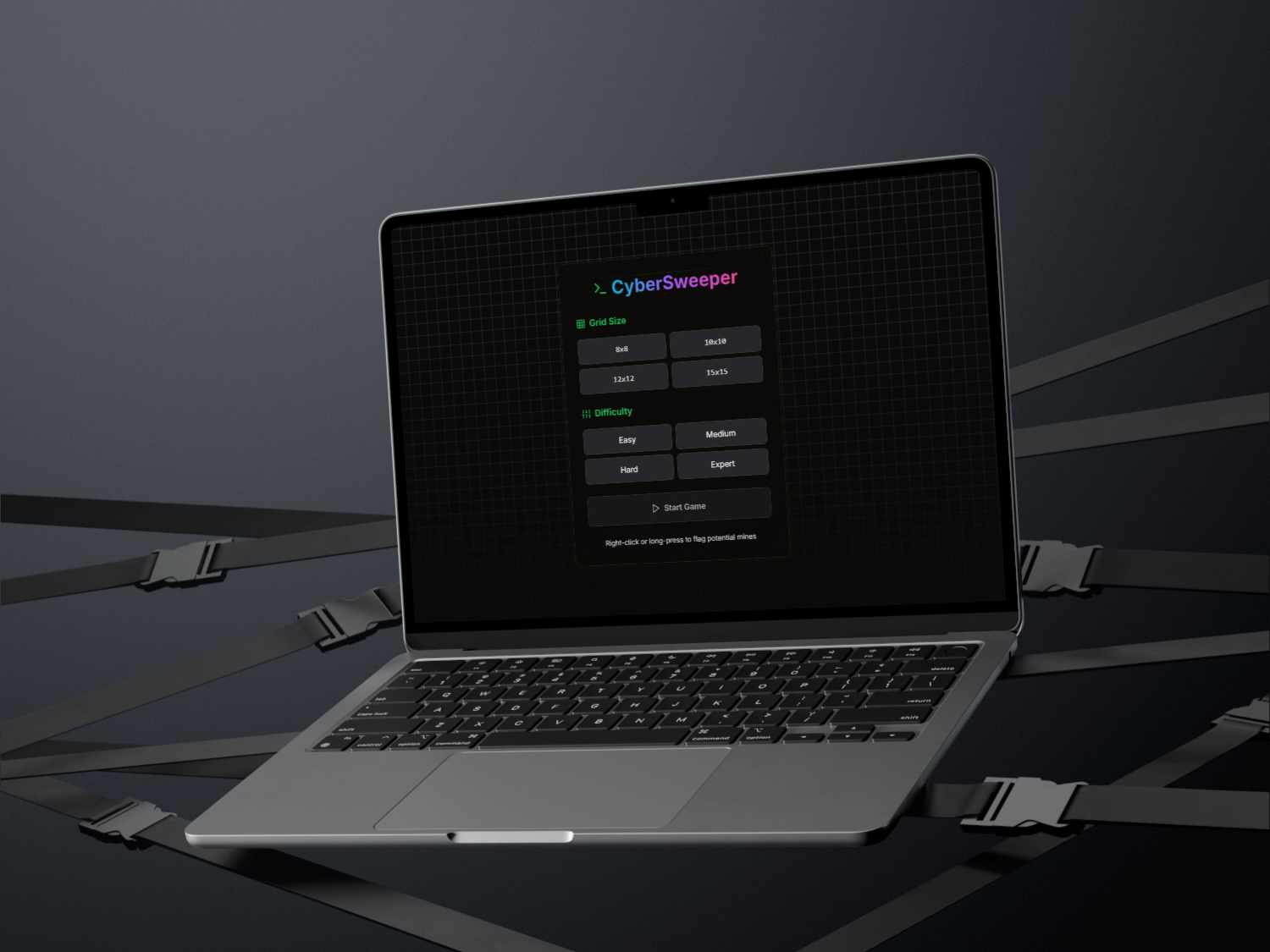
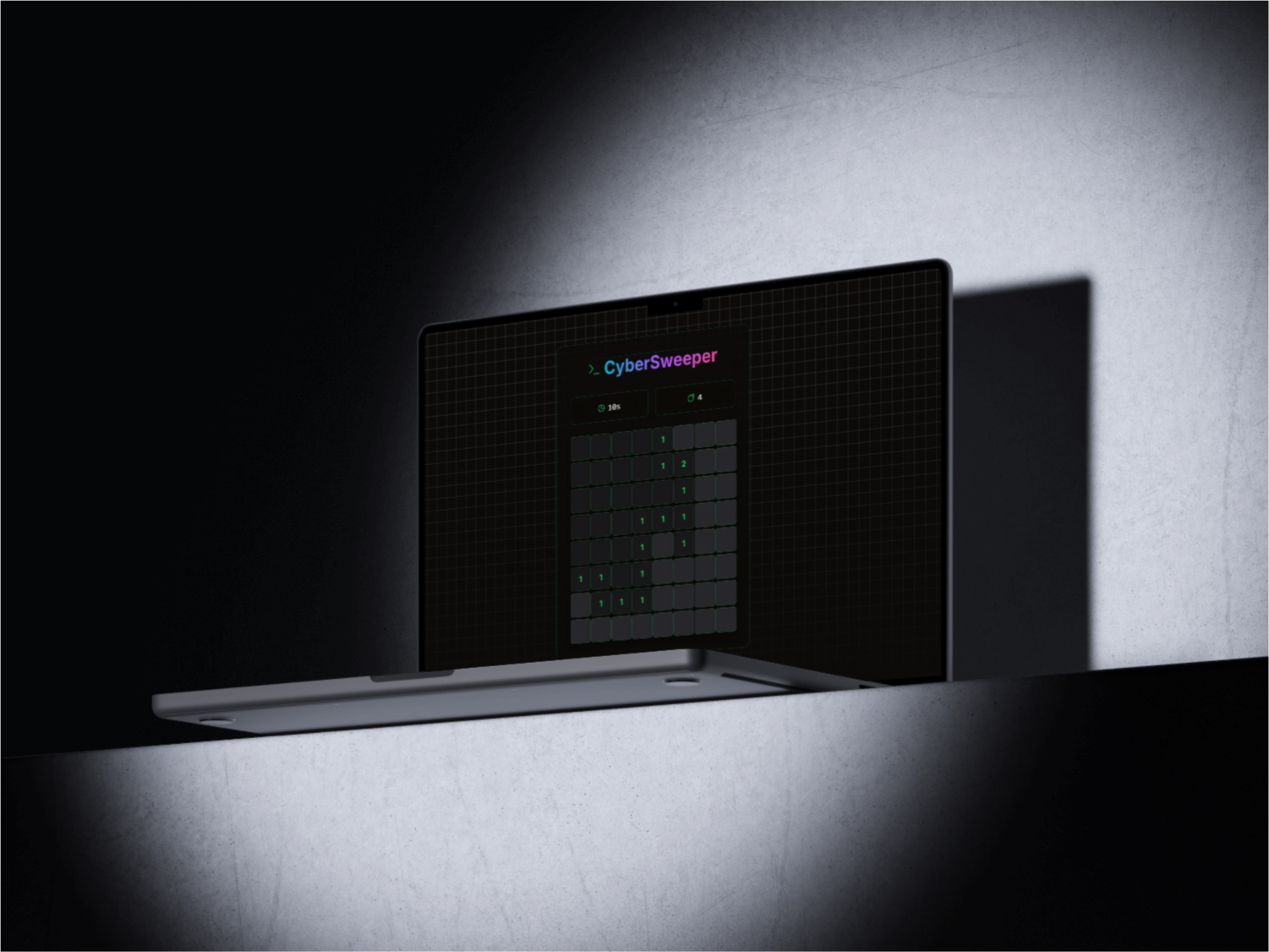
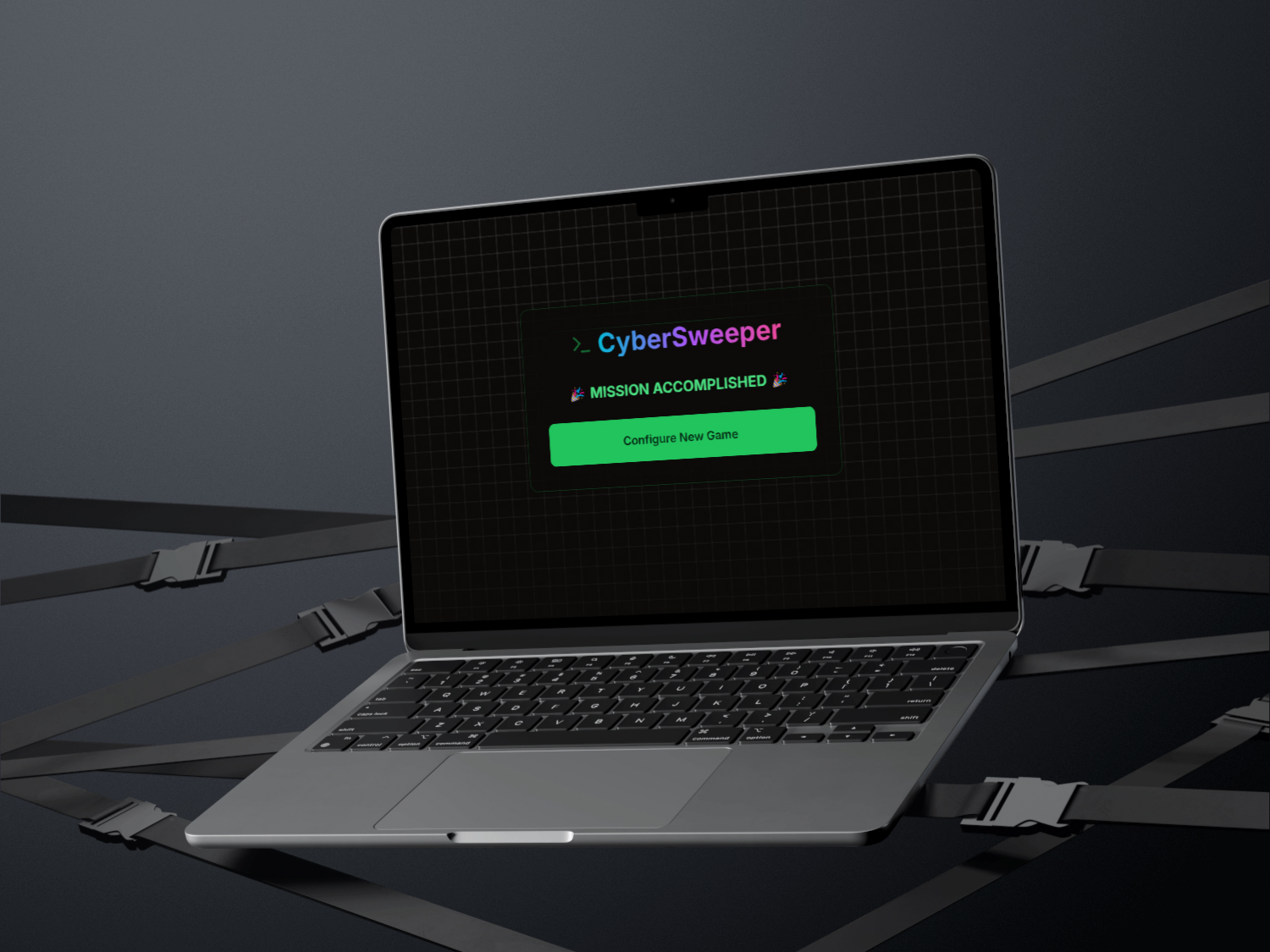
CyberSweeper
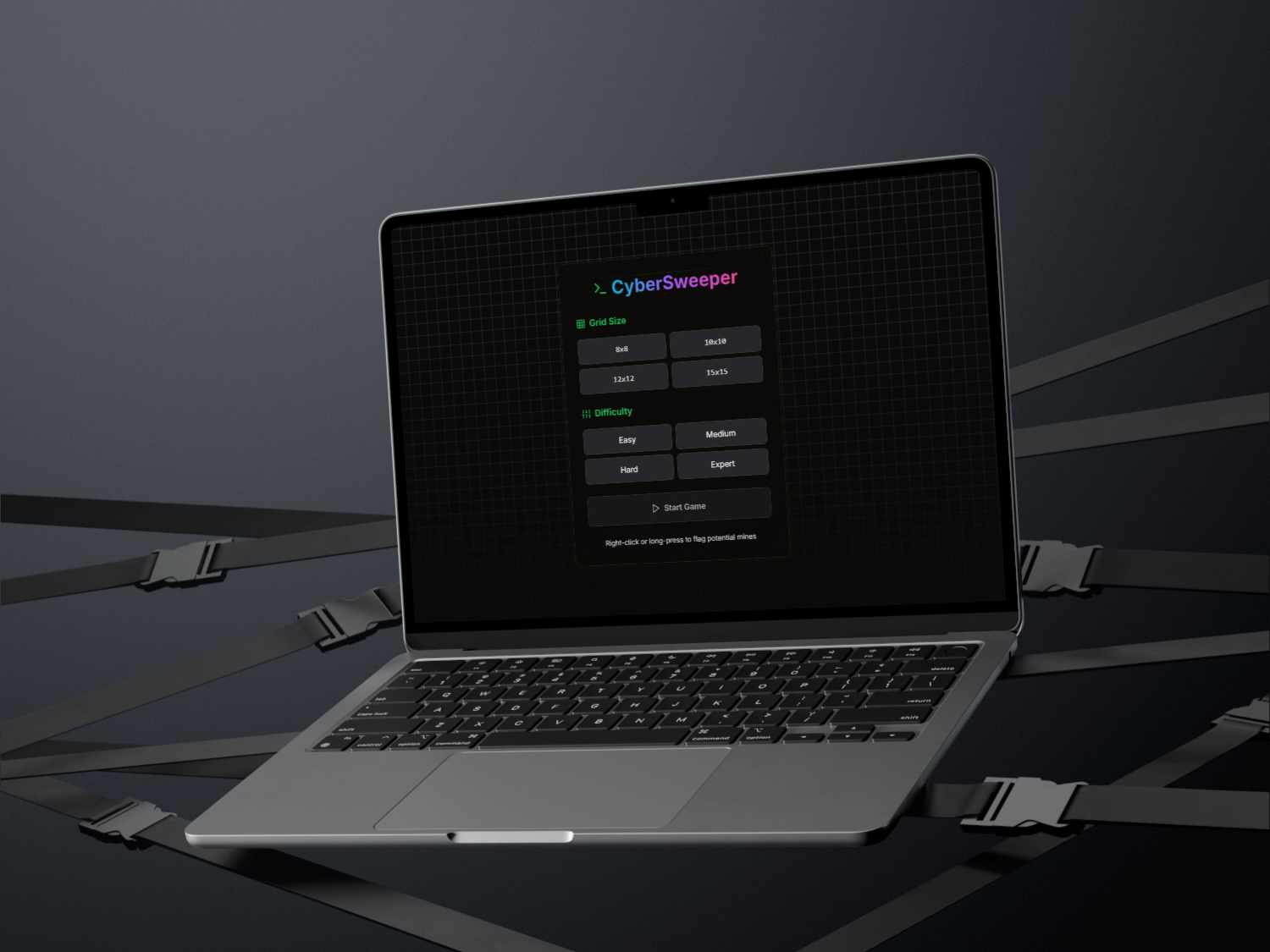
CyberSweeper 🎮
A modern, cyberpunk-themed take on the classic Minesweeper game, built with Next.js and TypeScript.
Features 🚀
- Modern Interface: Sleek, cyberpunk-inspired design with smooth animations
- Customizable Gameplay: Choose your grid size and difficulty level
- Responsive Design: Play seamlessly on any device
- Dark/Light Mode: Toggle between themes for optimal viewing
- Performance Optimized: Built with Next.js for lightning-fast gameplay
Tech Stack 💻
- Next.js - React framework
- TypeScript - Type safety
- Tailwind CSS - Styling
- Framer Motion - Animations
- Lucide Icons - Modern iconography
How to Play 🎲
-
Configure Your Game:
- Select grid size (8x8 to 15x15)
- Choose difficulty level (Easy to Expert)
-
Game Rules:
- Left-click to reveal cells
- Right-click to flag potential mines
- Clear all non-mine cells to win
- Avoid clicking on mines!
-
Difficulty Levels:
- Easy: 10% mines
- Medium: 15% mines
- Hard: 20% mines
- Expert: 25% mines
Features in Detail 🔍
Grid Sizes
- 8x8: Perfect for quick games
- 10x10: Classic minesweeper size
- 12x12: Enhanced challenge
- 15x15: Expert level
Game Statistics
- Real-time timer
- Click counter
- Win/loss tracking
UI/UX Features
- Smooth animations
- Responsive grid layout
- Cyberpunk theme
- Accessibility features
- Dark/light mode toggle
Technical Implementation
The game logic is implemented using TypeScript for type safety and better code organization. Here's a glimpse of the core game logic:
interface Cell {
isMine: boolean;
isRevealed: boolean;
isFlagged: boolean;
adjacentMines: number;
}
class GameBoard {
private grid: Cell[][];
private mineCount: number;
private size: number;
constructor(size: number, difficulty: Difficulty) {
this.size = size;
this.mineCount = this.calculateMineCount(difficulty);
this.grid = this.initializeGrid();
this.placeMines();
this.calculateAdjacentMines();
}
private initializeGrid(): Cell[][] {
return Array(this.size).fill(null).map(() =>
Array(this.size).fill(null).map(() => ({
isMine: false,
isRevealed: false,
isFlagged: false,
adjacentMines: 0
}))
);
}
private placeMines(): void {
let minesPlaced = 0;
while (minesPlaced < this.mineCount) {
const x = Math.floor(Math.random() * this.size);
const y = Math.floor(Math.random() * this.size);
if (!this.grid[y][x].isMine) {
this.grid[y][x].isMine = true;
minesPlaced++;
}
}
}
}
Development Process
The development of CyberSweeper followed these key steps:
-
Planning Phase
- Game mechanics design
- UI/UX wireframing
- Technology stack selection
-
Implementation
- Core game logic development
- UI component creation
- Animation system integration
-
Optimization
- Performance tuning
- Mobile responsiveness
- Accessibility improvements
-
Testing
- Cross-browser testing
- Mobile device testing
- User feedback integration
Future Enhancements
Planned features for future releases:
- Online multiplayer mode
- Global leaderboards
- Custom themes
- Achievement system
- Touch controls optimization
Contributing 🤝
Contributions are welcome! Please feel free to submit a Pull Request.
- Fork the project
- Create your feature branch
- Commit your changes
- Push to the branch
- Open a Pull Request
License 📄
This project is licensed under the MIT License.
Acknowledgments 🙏
- Inspired by the classic Minesweeper game
- Built with modern web technologies
Made with ❤️ by Justice Gooch
Project Gallery
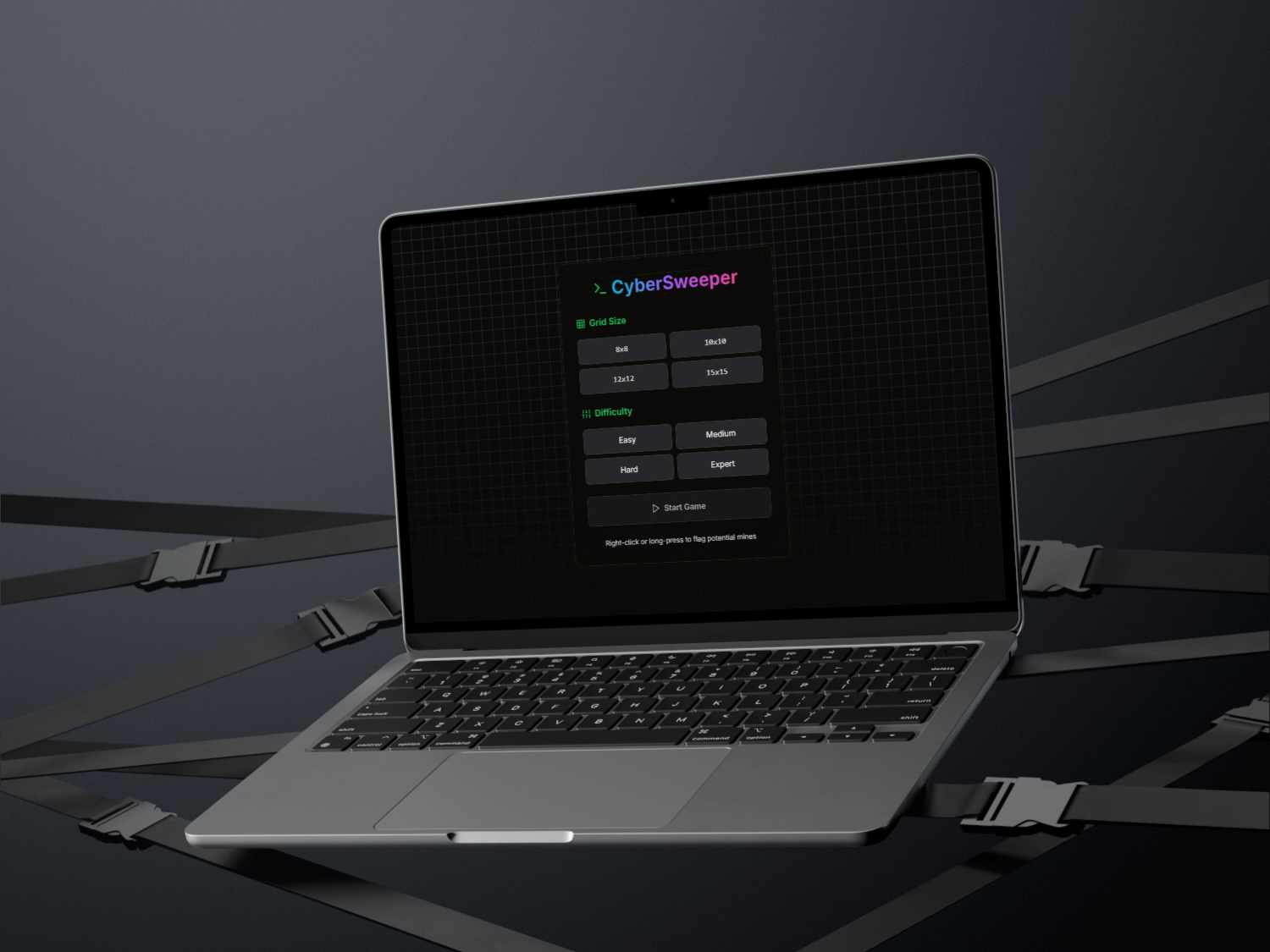
CyberSweeper Home Screen
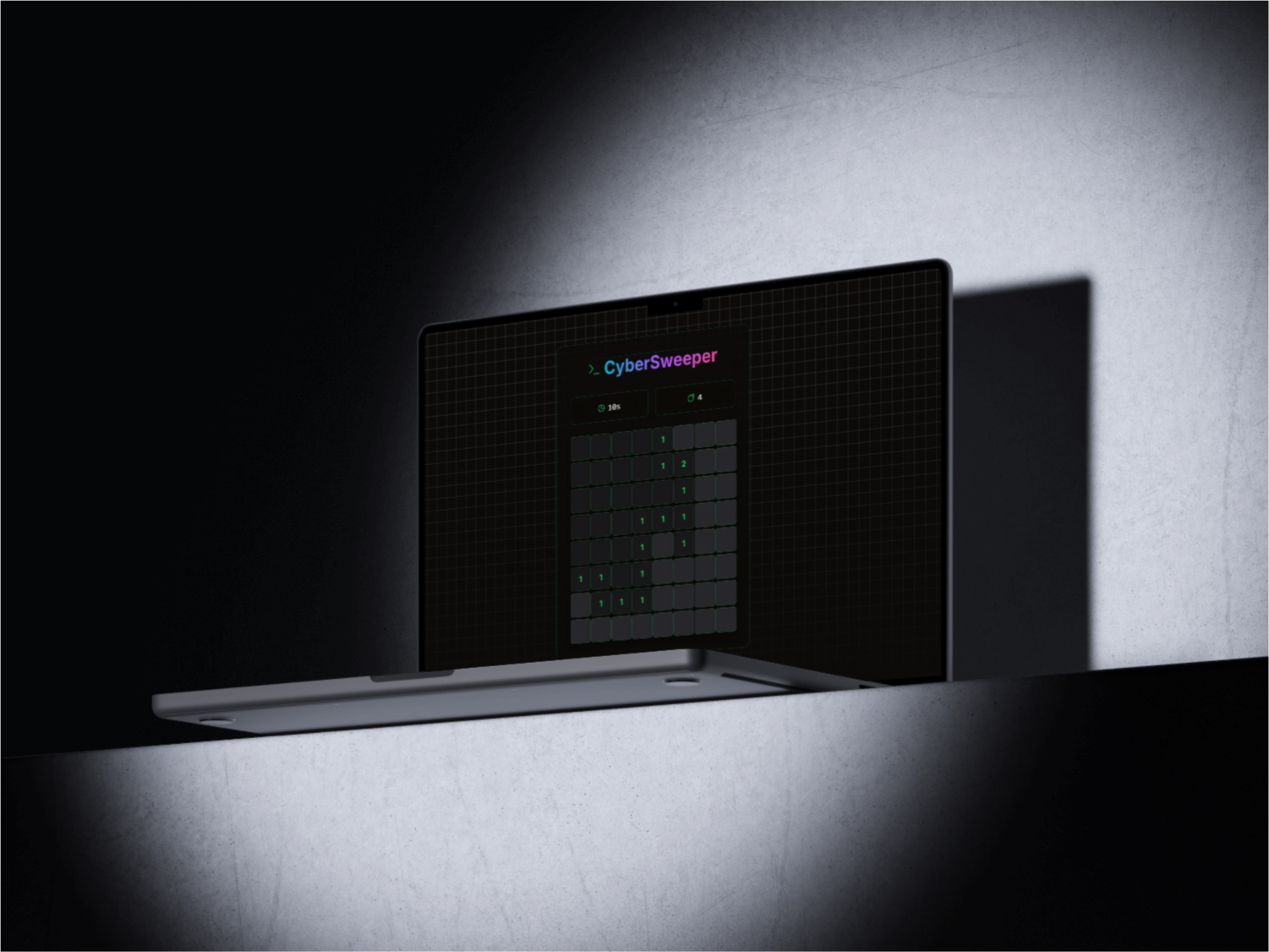
Gameplay Interface
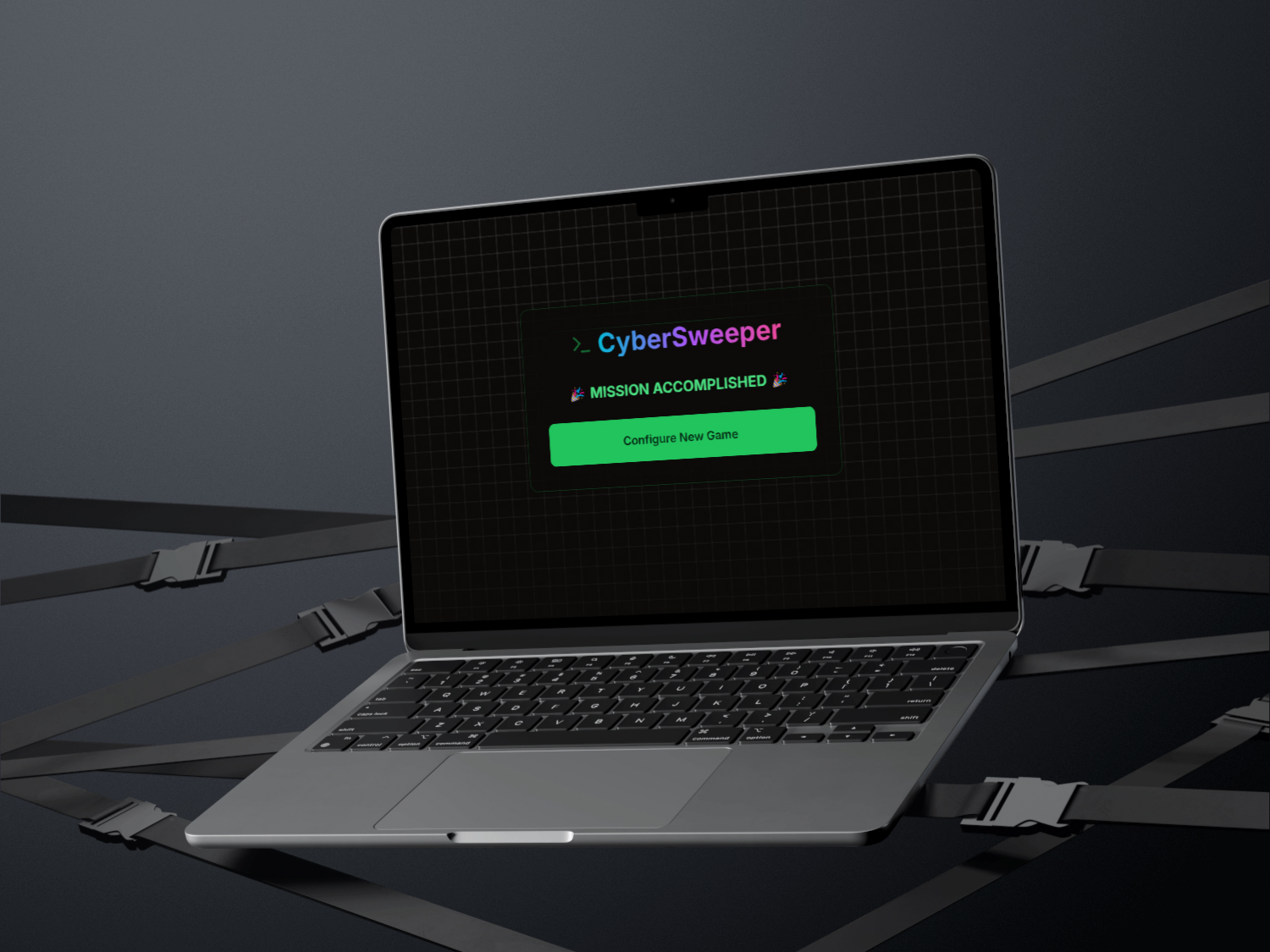
Victory Screen